HTML Structure
- Container: Holds the
login
andregistration
forms. - Forms:
- Login Form: Includes fields for email and password, a login button, social login buttons (Facebook, Google, GitHub), and a toggle link to switch to the registration form.
- Registration Form: Contains fields for full name, address, age, email, and password, a register button, and a toggle link to switch back to the login form.
- Background Element: Adds a gradient and animated glow effect behind the forms.
CSS Styling and Effects
- Basic Styles:
- A linear gradient background color and a radial gradient effect for the form background.
- Animated glow effect (
@keyframes glow
) that subtly changes the form container’s shadow and size. - The background glow animates between scales, adding to the immersive look.
- Form Styling:
- Smooth transitions and animations on focus states for input fields.
- Button transformations on hover, creating a glowing effect.
- Toggle links that switch between the forms with a fade animation.
- Social Login Buttons: Styled with colors for specific platforms and scale-up on hover.
- Responsive Design: The forms are centered and responsive to different screen sizes, with flexible max-widths.
JavaScript Functionality
- Form Toggle:
toggleForm()
: Toggles between displaying the login and registration forms, creating a seamless switch effect.
- Login Functionality:
- Validates the email and password input by checking against
localStorage
to see if there’s a match for an existing user. - If matched, a success message is displayed; if not, an error message appears.
- Validates the email and password input by checking against
- Registration Functionality:
- Validates fields to ensure all are filled and checks age for validity.
- Password Validation: Ensures it includes an uppercase letter, lowercase letter, number, and special symbol.
- Saves user data (name, address, age, email, password) to
localStorage
in lowercase for consistent comparison during login. - After successful registration, it displays a success message and toggles to the login form.
- Social Login Simulation:
socialLogin(platform)
: Simulates logging in with social media platforms with an alert, e.g., “Logging in with Facebook…”
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Modern Login & Registration</title>
<style>
/* Basic Reset */
* {
margin: 0;
padding: 0;
box-sizing: border-box;
}
body {
font-family: 'Roboto', sans-serif;
display: flex;
align-items: center;
justify-content: center;
height: 100vh;
background: linear-gradient(135deg, #1f4037, #99f2c8);
overflow: hidden;
color: #fff;
}
/* Form Background Glow */
.background {
position: absolute;
width: 100%;
height: 100%;
background: radial-gradient(circle, rgba(255, 204, 0, 0.15), rgba(0, 0, 0, 0.7));
animation: glow 20s infinite alternate;
z-index: 0;
}
@keyframes glow {
0% { transform: scale(1); }
50% { transform: scale(1.05); }
100% { transform: scale(1); }
}
/* Form Container */
.container {
position: relative;
width: 420px;
max-width: 95%;
padding: 2rem;
background: rgba(0, 0, 0, 0.75);
border-radius: 15px;
box-shadow: 0 0 30px rgba(0, 0, 0, 0.5);
overflow: hidden;
z-index: 1;
text-align: center;
transition: transform 0.5s ease, opacity 0.5s ease;
animation: glowing 1.5s infinite alternate;
}
@keyframes glowing {
0% {
box-shadow: 0 0 5px #ffdd57, 0 0 10px #ffdd57, 0 0 15px #ffdd57, 0 0 20px #ff8b00, 0 0 30px #ff8b00, 0 0 40px #ff8b00;
}
100% {
box-shadow: 0 0 10px #ffdd57, 0 0 20px #ffdd57, 0 0 30px #ffdd57, 0 0 40px #ff8b00, 0 0 50px #ff8b00, 0 0 60px #ff8b00;
}
}
.container h2 {
font-size: 2rem;
color: #ffdd57;
margin-bottom: 1.5rem;
font-weight: 700;
letter-spacing: 1px;
text-shadow: 0 0 8px rgba(255, 221, 87, 0.6);
}
.form {
display: none;
}
.form.active {
display: block;
animation: fadeIn 0.6s ease-in-out;
}
@keyframes fadeIn {
0% { opacity: 0; transform: translateY(10px); }
100% { opacity: 1; transform: translateY(0); }
}
/* Input Styling */
.input-group {
margin-bottom: 20px;
position: relative;
}
.input-group input {
width: 100%;
padding: 12px 16px;
font-size: 1rem;
color: #333;
background: rgba(255, 255, 255, 0.9);
border: none;
border-radius: 8px;
outline: none;
transition: box-shadow 0.3s ease;
}
.input-group input:focus {
box-shadow: 0 0 12px rgba(255, 221, 87, 0.7);
}
.input-group label {
position: absolute;
top: 50%;
left: 16px;
transform: translateY(-50%);
color: #666;
font-size: 0.9rem;
transition: all 0.3s ease;
pointer-events: none;
}
.input-group input:focus + label,
.input-group input:not(:placeholder-shown) + label {
top: -10px;
font-size: 0.75rem;
color: #ffdd57;
}
/* Buttons */
button {
width: 100%;
padding: 12px;
font-size: 1rem;
font-weight: bold;
color: #333;
background: linear-gradient(135deg, #fffefc, #000000);
border: none;
border-radius: 8px;
cursor: pointer;
transition: all 0.3s ease;
box-shadow: 0 0 15px rgba(255, 204, 0, 0.5);
margin-top: 10px;
}
button:hover {
transform: scale(1.05);
box-shadow: 0 0 20px rgba(255, 204, 0, 0.7);
}
.toggle-link {
margin-top: 1rem;
color: #ffdd57;
font-size: 0.9rem;
cursor: pointer;
transition: all 0.3s ease;
}
.toggle-link:hover {
text-shadow: 0 0 8px rgba(255, 204, 0, 0.8);
}
/* Social Login Buttons */
.social-login button {
padding: 10px;
margin: 0.5rem;
width: calc(33% - 1rem);
font-size: 0.9rem;
color: #fff;
border: none;
cursor: pointer;
border-radius: 8px;
transition: transform 0.3s;
}
.social-login button.facebook {
background-color: #4267B2;
}
.social-login button.google {
background-color: #DB4437;
}
.social-login button.github {
background-color: #000000;
}
.social-login button:hover {
transform: scale(1.1);
}
</style>
</head>
<body>
<div class="background"></div>
<div class="container">
<!-- Login Form -->
<div class="form active" id="login-form">
<h2>🌟 Login</h2>
<div class="input-group">
<input type="text" id="login-email" placeholder=" " required>
<label for="login-email">👤 Email</label>
</div>
<div class="input-group">
<input type="password" id="login-password" placeholder=" " required>
<label for="login-password">🔒 Password</label>
</div>
<button onclick="login()">Login</button>
<div class="social-login">
<button class="facebook" onclick="socialLogin('Facebook')">📘 Facebook</button>
<button class="google" onclick="socialLogin('Google')">📧 Google</button>
<button class="github" onclick="socialLogin('GitHub')">🐱 GitHub</button>
</div>
<p class="toggle-link" onclick="toggleForm()">Don’t have an account? Register here ➡️</p>
</div>
<!-- Registration Form -->
<div class="form" id="register-form">
<h2>🎉 Register</h2>
<div class="input-group">
<input type="text" id="reg-name" placeholder=" " required>
<label for="reg-name">📝 Full Name</label>
</div>
<div class="input-group">
<input type="text" id="reg-address" placeholder=" " required>
<label for="reg-address">🏠 Address</label>
</div>
<div class="input-group">
<input type="number" id="reg-age" placeholder=" " required>
<label for="reg-age">🎂 Age</label>
</div>
<div class="input-group">
<input type="email" id="reg-email" placeholder=" " required>
<label for="reg-email">📧 Email</label>
</div>
<div class="input-group">
<input type="password" id="reg-password" placeholder=" " required>
<label for="reg-password">🔒 Password</label>
</div>
<button onclick="register()">Register</button>
<p class="toggle-link" onclick="toggleForm()">Already have an account? Login here ➡️</p>
</div>
</div>
<script>
function toggleForm() {
const loginForm = document.getElementById('login-form');
const registerForm = document.getElementById('register-form');
loginForm.classList.toggle('active');
registerForm.classList.toggle('active');
}
function login() {
const email = document.getElementById('login-email').value.toLowerCase(); // Store email in lowercase
const password = document.getElementById('login-password').value;
const users = JSON.parse(localStorage.getItem('users')) || [];
const user = users.find(user => user.email === email && user.password === password);
if (user) {
alert("🎉 Login Successful! Welcome back! 🎈");
} else {
alert("🚨 Please enter correct information.");
}
}
function register() {
const name = document.getElementById('reg-name').value;
const address = document.getElementById('reg-address').value;
const age = document.getElementById('reg-age').value;
const email = document.getElementById('reg-email').value.toLowerCase(); // Store email in lowercase
const password = document.getElementById('reg-password').value;
if (!name || !address || !age || !email || !password) {
alert("🚨 Please complete all fields.");
return;
}
if (isNaN(age) || age <= 0) {
alert("🚨 Age should be a valid number.");
return;
}
const passwordPattern = /^(?=.*[a-z])(?=.*[A-Z])(?=.*\d)(?=.*[@$!%*?&])[A-Za-z\d@$!%*?&]{8,}$/;
if (!passwordPattern.test(password)) {
alert("🚨 Password must contain an uppercase letter, lowercase letter, number, and symbol.");
return;
}
// Store user data
const users = JSON.parse(localStorage.getItem('users')) || [];
users.push({ name, address, age, email, password });
localStorage.setItem('users', JSON.stringify(users));
alert("🎉 Registration Successful! 🎈");
toggleForm();
}
function socialLogin(platform) {
alert(`🚀 Logging in with ${platform}...`);
}
</script>
</body>
</html>
HTML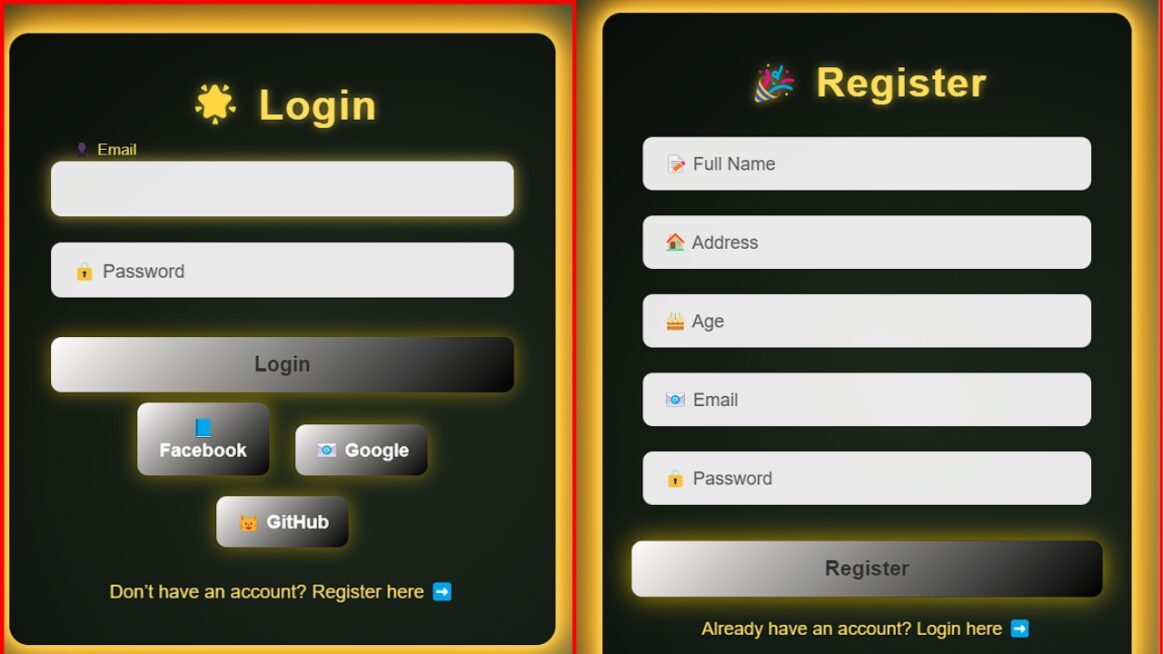
DOWNLOAD CODE
IF ANY FEEDBACK COMMENT DOWN