Introduction
Dart is a versatile programming language, widely used for building web, mobile, and desktop applications. One of the most basic and essential skills in programming is handling numbers and performing operations on them. In this program, we’ll demonstrate how to add five numbers in Dart. This will help you understand how to work with variables, arithmetic operations, and functions in Dart.
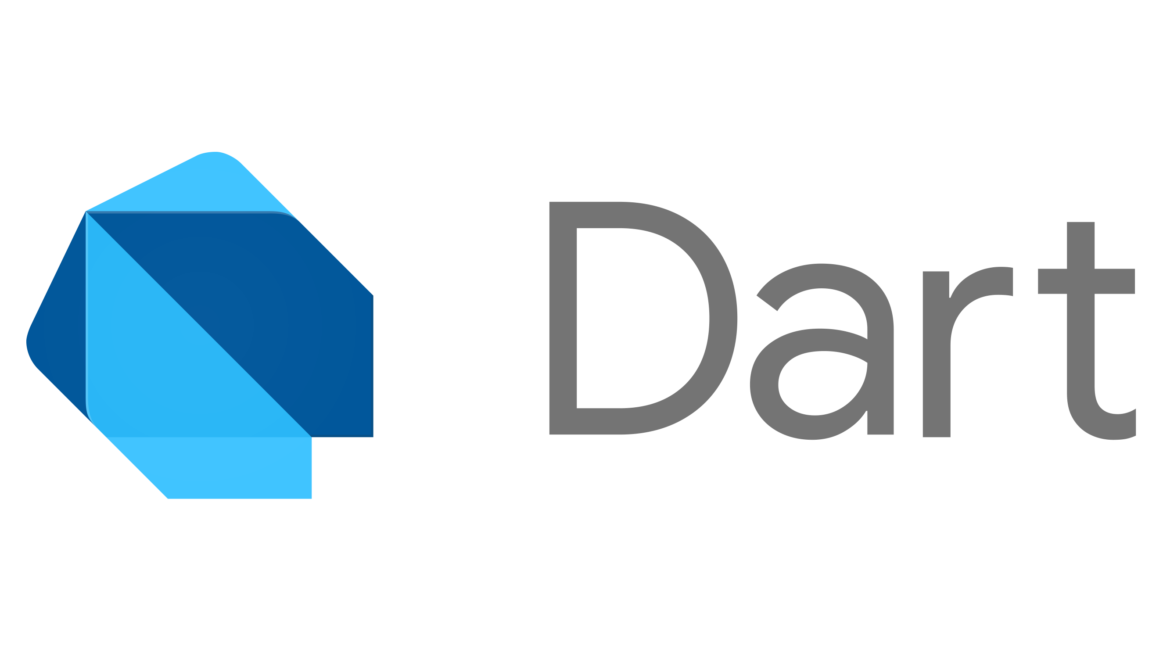
Dart Program to Add Five Numbers
void main() {
// Declaring five numbers
int num1 = 10;
int num2 = 20;
int num3 = 30;
int num4 = 40;
int num5 = 50;
// Adding the five numbers
int sum = num1 + num2 + num3 + num4 + num5;
// Printing the result
print("The sum of the five numbers is: $sum");
}
DartExplanation of the Code
void main()
: This is the entry point of the program where the execution begins.- Variable Declaration:
int num1 = 10;
– Here,int
stands for integer, and we are declaring five integer variables (num1
,num2
,num3
,num4
, andnum5
), each initialized with a number.
- Addition Operation:
int sum = num1 + num2 + num3 + num4 + num5;
– This line adds all five numbers and stores the result in the variablesum
.
- Output:
print("The sum of the five numbers is: $sum");
– Theprint
function outputs the result to the console, displaying the sum of the five numbers.
Output
When the program is executed, the output will be:
The sum of the five numbers is: 150
DartConclusion
This program demonstrates how to add five numbers using Dart. By declaring variables, performing arithmetic operations, and printing the result, you can efficiently handle numerical operations in Dart. This foundational knowledge is critical as you move on to more complex applications in Dart programming.