Introduction to Adding Numbers in Perl
Perl is a versatile programming language that is often used for tasks involving text manipulation, system administration, and scripting. However, it can also handle mathematical operations such as addition. In this tutorial, we will write a simple Perl program to add 5 numbers. We’ll explain each part of the code, how the addition works, and walk through the output.
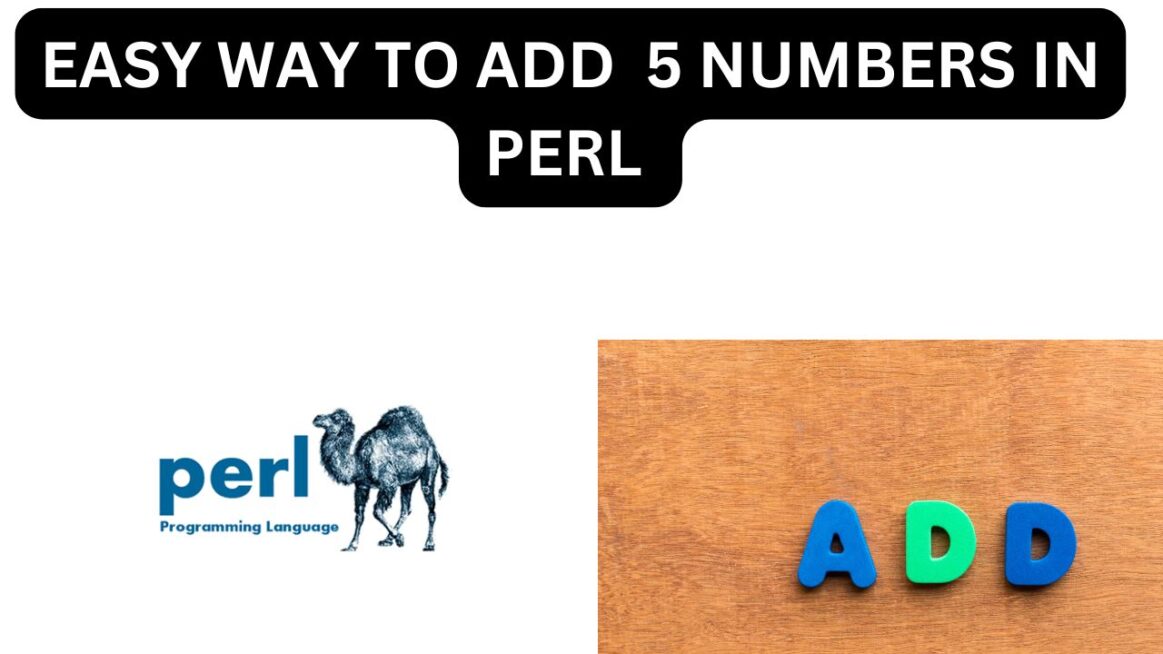
Perl Program to Add 5 Numbers
Here’s a simple Perl program that adds 5 numbers:
#!/usr/bin/perl
use strict;
use warnings;
# Declare and initialize the five numbers
my $num1 = 10;
my $num2 = 20;
my $num3 = 30;
my $num4 = 40;
my $num5 = 50;
# Add the numbers
my $sum = $num1 + $num2 + $num3 + $num4 + $num5;
# Print the result
print "The sum of the five numbers is: $sum\n";
PerlExplanation of the Code
Declaring and Initializing Variables
We declare 5 variables ($num1
, $num2
, $num3
, $num4
, and $num5
) and assign them values. These are the numbers we will add.
#!/usr/bin/perl
This is the shebang line, which tells the operating system where to find the Perl interpreter. It is important for running the script on Linux/UNIX systems but can be ignored on Windows.
use strict; use warnings;
These lines enable strict and warnings modes in Perl. This is good practice as it helps catch common programming mistakes like undeclared variables.
my $num1 = 10;
my $num2 = 20;
my $num3 = 30;
my $num4 = 40;
my $num5 = 50;
PerlAdding the Numbers
The addition operation is performed by summing all 5 variables and storing the result in the $sum
variable:
my $sum = $num1 + $num2 + $num3 + $num4 + $num5;
PerlPrinting the Result
Finally, we print the sum using the print
function:
print "The sum of the five numbers is: $sum\n";
PerlThe \n
at the end adds a new line after printing the output.
Output of the Program
When you run the program, the output will be:
The sum of the five numbers is: 150
PerlExplanation of the Output
The program adds the five numbers 10
, 20
, 30
, 40
, and 50
, which results in a sum of 150
. This sum is then printed on the screen using the print
function.
How to Run the Program
If you’re on Linux/UNIX, make the file executable:bash
Save the code in a file called add_numbers.pl
.
Open a terminal or command prompt.
chmod +x add_numbers.pl
PerlRun the program using:
./add_numbers.pl
PerlOn Windows, simply use:
perl add_numbers.pl
PerlConclusion
This simple Perl program demonstrates how to add numbers and output the result. Perl is an easy-to-learn yet powerful language, suitable for various tasks. By mastering basic operations like addition, you can go on to create more complex applications.
If you want to dive deeper into Perl programming, check out these helpful resources:
- Perl.org – The official Perl website.
- Learn Perl the Hard Way – A beginner-friendly resource.
- Modern Perl – Learn advanced Perl techniques for modern development.
Sites to Learn Perl:
Modern Perl
A free online book that covers modern Perl programming practices and best practices.
Perl.org
The official Perl website provides comprehensive documentation and resources to learn Perl.
Learn Perl the Hard Way
Offers a structured learning path for beginners with practical examples and exercises.
TutorialsPoint – Perl
Extensive tutorials with examples for beginners and advanced learners.
GeeksforGeeks – Perl Programming
Provides detailed guides, examples, and interview questions for learning Perl.