Introduction:
In this program, we will calculate the factorial of a given number. A factorial of a non-negative integer nnn, denoted as n!n!n!, is the product of all positive integers less than or equal to nnn. Factorials are used in various fields like mathematics, statistics, and computer science.
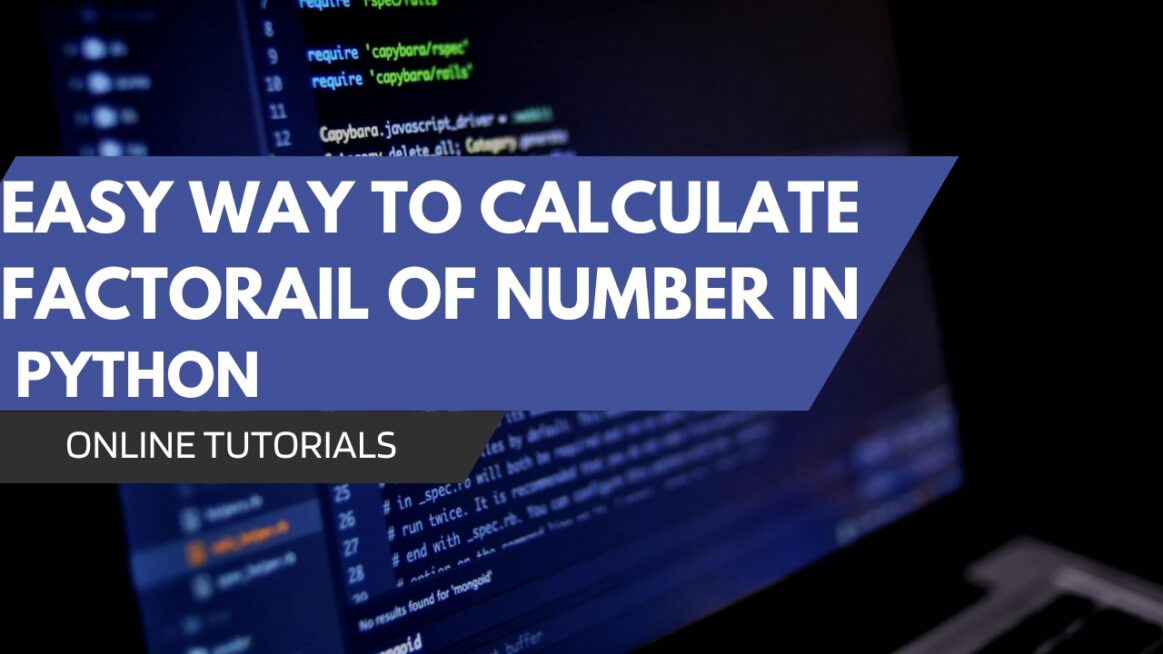
Python Program to Calculate Factorial:
# Introduction to the program:
# This program calculates the factorial of a given number entered by the user.
# Function to calculate factorial of a number using recursion
def factorial(n):
# Base condition: factorial of 0 or 1 is 1
if n == 0 or n == 1:
return 1
else:
# Recursive case: n * factorial(n-1)
return n * factorial(n - 1)
# Input: Asking the user to enter a non-negative integer
n = int(input("Enter a non-negative integer: "))
# Output: Checking if the number is non-negative and calculating the factorial
if n < 0:
print("Factorial is not defined for negative numbers.")
else:
print(f"The factorial of {n} is: {factorial(n)}")
PythonExplanation of the Syntax:
Function Definition:
def factorial(n):
Pythondef
is used to define a function named factorial
.
n is the argument passed to the function, which represents the number for which the factorial will be calculated.
Base Condition:
if n == 0 or n == 1:
return 1
PythonThe base condition checks if the input is 0
or 1
. The factorial of 0
and 1
is 1
, so the function returns 1
.
Recursive Case:
else:
return n * factorial(n - 1)
PythonIf n
is greater than 1
, the function calls itself recursively with n - 1
. The product of n
and factorial(n - 1)
gives the factorial of n
.
User Input:
n = int(input("Enter a non-negative integer: "))
Pythoninput()
prompts the user to enter a number.
int()
converts the input into an integer, since input()
returns a string by default.
Condition to Check Negative Input:
if n < 0:
print("Factorial is not defined for negative numbers.")
PythonThis if
statement ensures that the user doesn’t enter a negative number. If they do, a message is displayed saying that factorials are not defined for negative numbers.
6.Printing the Result:
print(f"The factorial of {n} is: {factorial(n)}")
PythonThe print()
function displays the result.f"The factorial of {n} is: {factorial(n)}"
is an f-string used to format the output, where {n}
is the number entered by the user, and {factorial(n)}
is the result of the function.
Sample Output:
Enter a non-negative integer: 5
The factorial of 5 is: 120
PythonConclusion:
In this program, we explored how to calculate the factorial of a number using recursion. The program ensures that the user enters a non-negative integer and handles negative inputs by displaying an appropriate message. We used recursion to simplify the process of calculating the factorial, which is a great example of breaking down a complex problem into smaller sub-problems.
This program introduces fundamental concepts of recursion, function definitions, and conditional statements in Python.
Official Python Documentation
- Overview: This is the official Python documentation provided by Python.org. It’s a comprehensive resource that includes Python tutorials, libraries, modules, and in-depth explanations of Python syntax and built-in functions.
- Why Visit: Best for understanding core concepts, syntax, and Python library usage. Suitable for both beginners and advanced learners.
2. W3Schools – Python Tutorial
- Overview: W3Schools is a well-structured website offering tutorials on Python with examples and interactive code editors.
- Why Visit: It’s beginner-friendly, and you can practice writing Python code directly in your browser. Covers basic to intermediate Python programming.
3. Real Python
- Overview: Real Python offers high-quality Python tutorials on a wide range of topics, from beginner to advanced level, including web development, automation, and data science.
- Why Visit: Offers both text-based and video tutorials, covering real-world applications of Python. Ideal for hands-on learning and practical usage.
4. GeeksforGeeks – Python Programming Language
- Overview: GeeksforGeeks provides a vast collection of Python tutorials, examples, and programming challenges.
- Why Visit: It’s great for learners who want to go in-depth on Python algorithms, data structures, and interview preparation.
5. Python for Beginners
- Overview: This website offers tutorials specifically aimed at Python beginners. It has easy-to-follow guides and projects that are great for building foundational Python skills.
- Why Visit: It’s simple and straight to the point, focusing on beginners who want to quickly get started with Python programming.
6. Programiz – Learn Python
- Overview: Programiz offers Python tutorials from the ground up, with a focus on building fundamental programming skills.
- Why Visit: It’s beginner-friendly and allows you to practice code in their online Python compiler. Covers topics from basic to advanced Python.
7. Codecademy – Learn Python 3
- Overview: Codecademy offers an interactive course on Python 3, with real-time feedback as you write code.
- Why Visit: Ideal for those who prefer an interactive learning approach. It’s structured, making it easy to follow for beginners.
8. Coursera – Python for Everybody
- Overview: This is a popular specialization offered by the University of Michigan. It provides comprehensive courses that take learners from beginners to intermediate-level Python programming.
- Why Visit: Great for learning with structured courses, quizzes, and assignments. Coursera offers certification for completing courses.
9. Kaggle Learn – Python
- Overview: Kaggle is known for its data science competitions, but it also offers free Python courses focused on data science and machine learning.
- Why Visit: Ideal for those interested in Python for data analysis, machine learning, and artificial intelligence.
10. LearnPython.org
- Overview: A free and interactive Python tutorial website that allows you to practice Python code within your browser.
- Why Visit: Beginner-friendly with an interactive platform. It’s a good resource for quick practice and learning Python fundamentals.
These resources cater to different levels of Python learners, from absolute beginners to more advanced users interested in specialized applications of Python such as web development, automation, and data science.