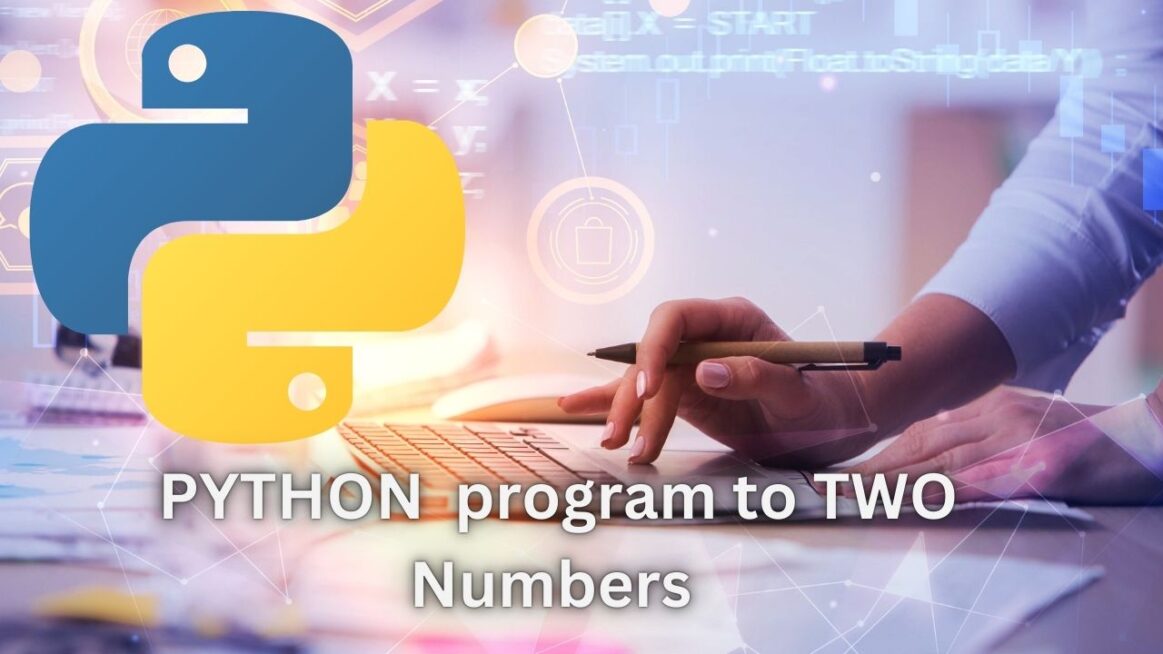
Introduction
Adding two numbers is one of the most fundamental operations in programming, often used to illustrate the basic syntax and structure of a programming language. This simple task helps us understand how different languages handle variables, functions, and output. Below, we’ll explore the basic code for adding two numbers in various programming languages and break down the core components of each example to make the concept clear.
Code
# Python code to add two numbers
def add_numbers(a, b):
return a + b
# Example usage
num1 = 5
num2 = 10
result = add_numbers(num1, num2)
print("Sum:", result)
Python-
EXPLANATION
-
Define the Function
- In Python, functions are defined using the
def
keyword. Here’s how you define a function to add two numbers:pythondef add_numbers(a, b): return a + b
def
indicates the start of a function definition.add_numbers
is the name of the function.(a, b)
are parameters that the function will accept.return a + b
computes the sum ofa
andb
and returns the result.
- In Python, functions are defined using the
-
Set Up Input Values
- To use the function, you need to specify the values you want to add. In the example:
python
num1 = 5 num2 = 10
num1
andnum2
are variables that store the numbers you want to add. Here,num1
is set to 5, andnum2
is set to 10.
- To use the function, you need to specify the values you want to add. In the example:
-
Call the Function
- After defining the function and setting the values, you call the function to get the result:
python
result = add_numbers(num1, num2)
add_numbers(num1, num2)
executes the function withnum1
andnum2
as arguments.- The returned value (sum of 5 and 10) is stored in the variable
result
.
- After defining the function and setting the values, you call the function to get the result:
-
Print the Result
- To display the result, use the
print
function:pythonprint("Sum:", result)
print
outputs the string"Sum:"
followed by the value ofresult
(which is 15).
- To display the result, use the
-
Run the Code
- To see the output, save the code in a file, for example,
add.py
, and run it using:shpython add.py
- This command executes the Python script and displays the output in the terminal or command prompt.
- To see the output, save the code in a file, for example,
-
Understand the Output
- When you run the script, the output will be:
makefile
Sum: 15
- This confirms that the function correctly added the numbers 5 and 10, displaying the sum as 15.
- When you run the script, the output will be:
Usage: Save the code in a file named add.py
. Run it using the command:
sh
python add.py
OUTPUT
SEE THE OUTPUT OF THE ABOVE CODE
Sum: 15
PythonSEE COMPLETELY COMPILED CODE
PREES PLAY BUTTON TO RUN THE PROGRAM
Conclusion
The Python code for adding two numbers showcases the language’s simplicity and effectiveness. By defining a function, setting input values, calling the function, and printing the result, you can perform basic arithmetic operations easily. Python’s clear syntax makes it a great choice for learning programming fundamentals. For more information on Python functions and basic operations, you can visit the official Python documentation.
COMPILE CODE : CODE COMPILER